
JavaScript code to create a speech recognition app for ChatGPT?
To create an app with voice recognition, you can use the Web Speech Recognition API, available in modern browsers. Here is an example of code in HTML and JavaScript to create a simple app that recognizes the user's voice and displays the transcribed text:
htmlCopy code
<!DOCTYPE html>
<html>
<head>
<title>Riconoscimento Vocale</title>
</head>
<body>
<h1>Riconoscimento Vocale</h1>
<button id="start-recognition">Avvia Riconoscimento</button>
<div id="transcription"></div>
<script>
// Controlla se il browser supporta l'API di riconoscimento vocale
if ('webkitSpeechRecognition' in window) {
const recognition = new webkitSpeechRecognition();
// Imposta alcune opzioni per il riconoscimento
recognition.continuous = true;
recognition.interimResults = true;
recognition.lang = 'it-IT'; // Puoi impostare la lingua desiderata
const startButton = document.getElementById('start-recognition');
const transcription = document.getElementById('transcription');
startButton.addEventListener('click', function() {
startButton.disabled = true;
recognition.start();
});
// Gestisci i risultati del riconoscimento
recognition.onresult = function(event) {
const result = event.results[event.results.length - 1];
const transcript = result[0].transcript;
transcription.innerHTML = transcript;
};
// Gestisci gli errori
recognition.onerror = function(event) {
console.error('Errore di riconoscimento vocale:', event.error);
};
// Termina il riconoscimento quando si preme il pulsante di avvio nuovamente
recognition.onend = function() {
startButton.disabled = false;
};
} else {
alert('Il tuo browser non supporta l\'API di riconoscimento vocale.');
}
</script>
</body>
</html>
In this example, we have a button “Start Recognition” which launches the speech recognition API when pressed. The transcribed text will appear in the item <div>
con id “transcription”. You can customize the language by setting recognition.lang
on the desired language.
Make sure you use this code in an environment with support for the Web Speech Recognition API, like a modern browser. Please note that user experience may vary depending on your browser and selected language.
Which browsers is this example compatible with??
The speech recognition example provided in my previous message uses the API webkitSpeechRecognition
, which is specific to the Chrome browser. Therefore, this specific example will only be compatible with the Google Chrome browser.
However, It is important to note that compatibility with the speech recognition API varies between browsers and versions. To ensure compatibility with a wider range of browsers, you can use the standard speech recognition API (SpeechRecognition
) rather than webkitSpeechRecognition
. Here's an example of how you can modify the code to use the standard API:
javascriptCopy code
if ('SpeechRecognition' in window || 'webkitSpeechRecognition' in window) {
const SpeechRecognition = window.SpeechRecognition || window.webkitSpeechRecognition;
const recognition = new SpeechRecognition();
// ...
}
Using window.SpeechRecognition || window.webkitSpeechRecognition
, you can support browsers that use the standard API (for example, Firefox) and those using the webkit prefix version (come Chrome).
However, Please note that API implementation and language support may vary from browser to browser. Therefore, It is important to carefully test speech recognition in different browsers to ensure the best possible user experience.
Which browsers support SpeechRecognition?
In September 2021, the speech recognition API (SpeechRecognition
) was supported by several browsers, but specific support may vary by browser and platform. Below are some of the major browsers that supported this API:
- Google Chrome: Chrome has good support for the speech recognition API. The API was introduced in Chrome 25 and support has been steadily improved over time.
- Mozilla Firefox: Firefox started supporting the speech recognition API starting with the release 44. However, support may require manual activation in your browser settings.
- Microsoft Edge: Microsoft Edge has also started supporting the speech recognition API. Support may vary depending on browser versions.
- Safari: Apple's Safari supports speech recognition API on platforms like iOS.
- Opera: Opera also offers support for the speech recognition API, but specific support may vary by version.
It is important to note that specific language and feature support may vary between browsers. In addition, Mobile browsers like Chrome and Safari often support speech recognition API on mobile devices.
Since browser support may change over time with the introduction of new versions and standards, I recommend that you check your browser documentation and carefully test the API on the specific browsers you intend to support.
ABOUT US
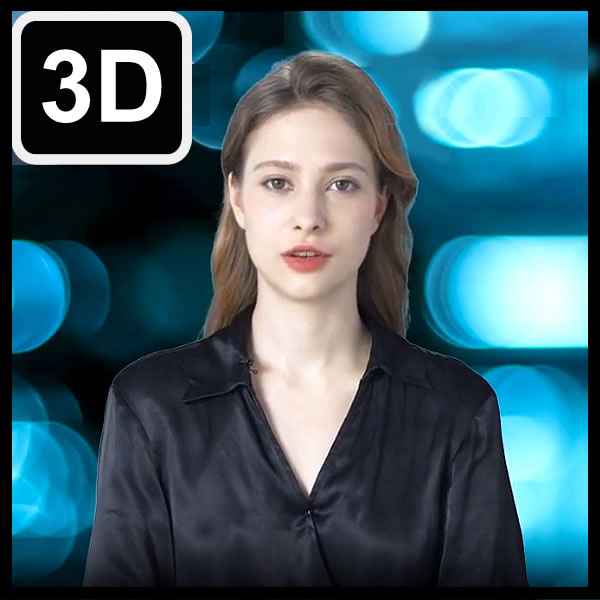